Posted At: Feb 29, 2024 - 759 Views
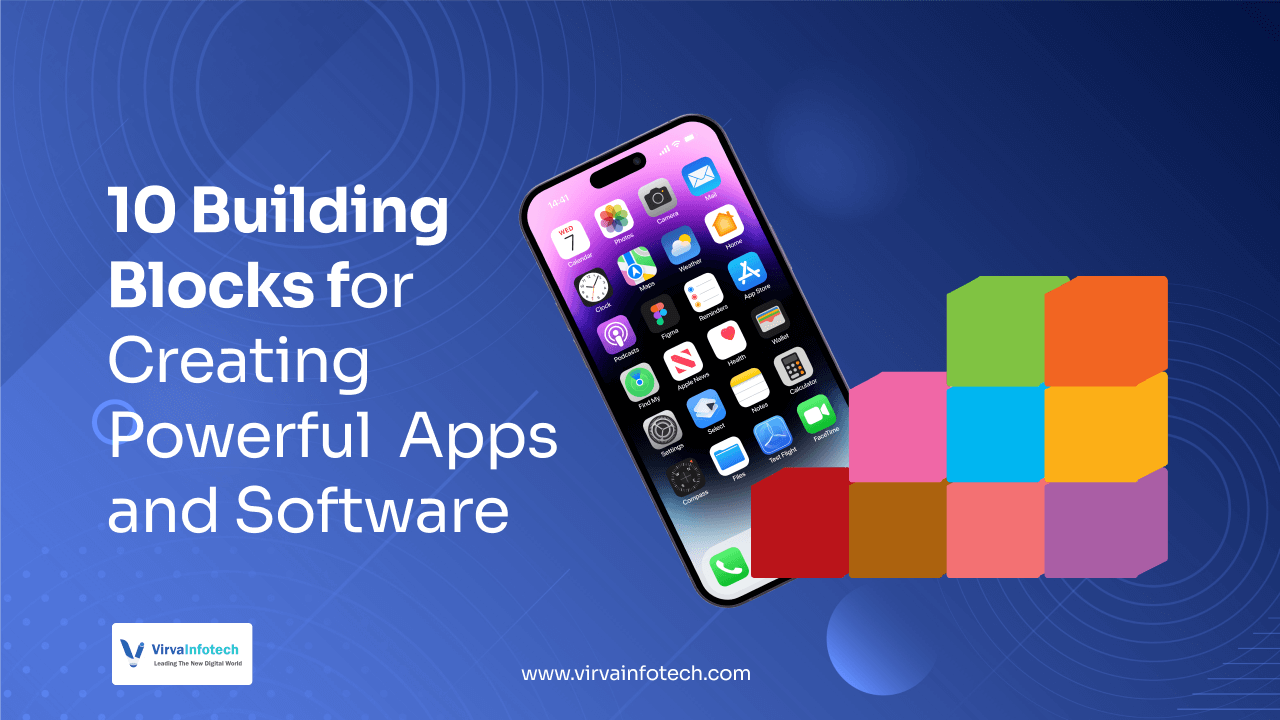
Essentially, keeping ahead in software development requires one to be constantly unschooled in mastering the core skills. If you are a novice beginner getting in touch with code or a developed person sharpening his skills, then you will definitely want to learn these 10 fundamental coding skills to help you create efficient, scalable, and easy-to-use applications. Knowing and understanding about data structures and algorithms, UI/UX design, and security practices are what makes the combination of these skills the backbone of the toolkit of a successful developer. After which we will cover the most critical concepts every programmer should have, practically explained and with links to get you started. Let the diving begin!
1. Data Structures and Algorithms
Data structures are the very backbone of software development: organizing, when retrieving, and managing data. They are similar to filing systems: optimizing how data is stored and accessed.
Key data structures : Arrays, Linked Lists, Tree, Hash Tables, Graphs.
Importance:
Efficient data storage and retrieval: Choosing the right data structure will determine the time complexity and space complexity of the operation.
Scalability: Proper classification allows data to be correctly managed, despite the increase in the volume of data over time.
Algorithms are basically stepwise instructions used in manipulating data in these structures. Searching, sorting and graph traversal algorithms are some of the generic algorithms that do the work of indirectly solving a problem.
Why Algorithms Matter:
Optimization: Efficient algorithms ensure that even when the data size increases, software works efficiently on it.
Problem Solving: A very important feature is that coding challenges are solved using algorithms. It improves one's logical thinking.
Resources to Learn:
GeeksforGeeks - Data Structures & Algorithms Tutorial
LeetCode - Coding Practice
2. Object-Oriented Programming (OOP)
One has got Open Object Programming along with its major four concepts through which creation becomes modular and structured in software development.
Classes: These are the blueprints that specify the properties and methods.
Objects: The instantiated classes are used for abstracting data and behaviour.
Inheritance: Code reusability is enabled because new classes can inherit properties from existing classes.
Polymorphism: This implies that a single interface can mean different types hence giving flexibility.
Benefits of OOP:
Modular Code: Maintains and debugs easily.
Scalable Applications: Fits into an enormous and most complicated system.
Reusability: Reduces code duplication.
For instance, one may create a Car class as blueprints to various objects like Sedan or ElectricCar. Inheriting some properties, for example, battery capacity could be defined for electric cars.
Resources to Learn:
Java Tutorial: Object-Oriented Programming
3. Software Design Principles
Software design principles serve one great purpose, that is, the building of applications that are robust, maintainable, and scalable. The most prominent of these principles is the following SOLID principles:
Single Responsibility Principle: A class should have only one reason to change.
Open-Closed Principle: Classes should be open for extension but closed for modification.
Liskov Substitution Principle: Substituted classes should be replaceable without altering the correctness of the program.
Interface Segregation Principle: Clients should not be forced to depend on interfaces they do not use.
Dependency Inversion Principle: Depend upon abstractions, not concretions.
Why It Matters:
Improve and clean your code.
Maintain it for a long period.
Reduce project complexity.
Resources to Learn:
Uncle Bob’s Blog - SOLID Principles
4. Version Control Systems (VCS) like Git
As a Version Control System, Git is the essential tool for managing changes made to code bases being created.
Key Features of Git:
Version Tracking: Allows tracking of all changes done by users.
Branching and Merging: Enable users to work in isolation on feature additions or bug fixes, and then merge it with the trunk when it is complete.
Collaboration: Multiple developers are now enabled to work simultaneously.
Benefits:
Effectively records code changes.
Helps the team in proper collaboration.
Version history prevents loss of data.
Resources to Learn:
Git SCM Documentation
GitHub Learning Lab
5. Databases and SQL
A strong grasp of databases and SQL (Structured Query Language) is essential for managing application data.
Relational Databases: Tables are used to store interrelated data (e.g., MySQL, PostgreSQL)
Commands in SQL: Commands for carrying operations with SELECT, INSERT, UPDATE, and DELETE.
Data Modeling: Designing efficient database architectures using the correct relationships.
Why It Matters:
Data Management: To store and retrieve data efficiently.
Optimized Queries: This leads to finely written SQL to boost performance.
Resources to Learn:
MySQL Tutorial
W3Schools SQL Tutorial
6. Programming Languages
Mastering one or more coding languages is an essential part of being a developer.
Here are some of the popular programming languages:
Python: Very versatile and easy to learn, it is most commonly used for web development and data science.
Java: The most widely used language for Android development and Enterprise applications.
JavaScript: The literal backbone of any front-end and back-end development (Node.js).
C++/C#: High-performance applications and game development.
Key to Success:
Understand native language syntax and libraries.
Keep abreast with frameworks and best practices.
7. Testing and Debugging
Testing ensures the functionality of the software, whereas debugging is the identification and resolution of problems.
Types of Test:
Unit Testing: Testing individual units.
Integration Testing: Inter-component behavioral verification.
System Testing: Full program evaluation.
Why Testing Matters:
Ensures software quality.
Reduces bugs in production.
Improves the user experience.
Tools: JUnit, Selenium, Py Test, Postman.
8. UI/UX Design Principles
A perfectly designed app encompasses a utility along with its aesthetics, where its simplicity combines with intuitive design. Modern UI/UX design purposely aims to create user-comfortable interfaces.
Core Concepts:
Layout and Navigation: A clear structure for easy interaction.
Visual Hierarchy: Prioritize Important Content.
Consistency: Maintain design standards.
Responsiveness: Optimize for All Devices.
Resources:
Interaction Design Foundation
Nielsen Norman Group
9. Security Best Practices
In today’s digital landscape, ensuring application security is critical to protect user data.
Key Practices:
Safe coding: Don't allow any vulnerabilities in programming such as SQL injection or cross-site scripting.
Efficient authentication: Establish strong user authentication systems.
Data Encryption: Encrypt confidential information while it is in transit or at a secure location.
Resources to Learn:
OWASP Top 10 Security Risks
SANS Institute
10. Software Development Methodologies
Different methodologies of development increase action efficacy as a whole in the project.
Key Approaches:
Agile: Iterative development with frequent review of progress.
Waterfall: A sequential methodology that is best applied to projects that have a clear definition.
Lean: Focuses on reducing waste and optimizing efficiency.
Why It Matters:
Enables collaboration among the team.
It aligns development with business goals.
Resources:
Agile Software Development
Waterfall Model