Posted At: Oct 12, 2023 - 1,230 Views
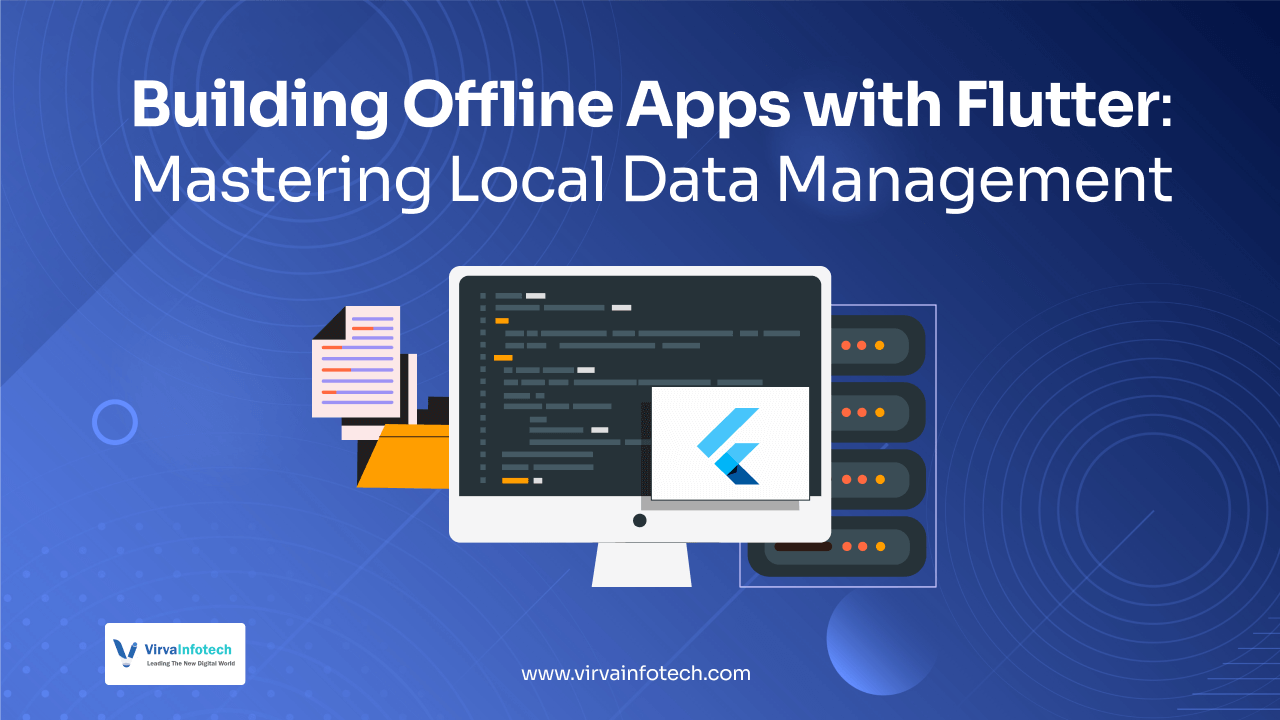
A long-awaited scene for the mobile applications today is that it should present a good user experience with or without an active internet connection. Many users are quite disappointed with an app that can be impaired by failing connectivity. Unfortunately, problems with networks, bandwidth, and other forms of connectivity may lead to such cases. The offline-first app may well solve this problem. It allows core features and content to be accessed by the user without a stable internet connection for continued use without interruption.
By using Google's powerful cross-platform UI toolkit, codenamed Flutter, you can build applications that are rich and robust offline; all you need are its fabulous libraries and tools to do the job. Good local data management strategies taken into consideration at the developmental stage could enable the application to work efficiently both online and offline. In this guide, we will present different techniques, tools, and best practices to be followed while building offline apps on Flutter.
Why Build Offline-First Apps?
Now let's review some critical reasons why offline-first apps have great importance these days before getting started with the implementation. User Convenience: Users, by all means, expect their apps to work at any time, regardless of conditions that are imposed on the network.
1. User Convenience: Applications for users should run at all times irrespective of network connectivity.
2. Improved User Experience: Offline applications help minimize latency, cause speed improvements, and a quicker response interface.
3. Accessibility: Now these applications can also reach inaccessible areas due to lack of or little internet connectivity.
4. Reliability of Data: Caching data locally ensures disruption-free work for users.
5. Higher Retention: To be able to use offline features, user satisfaction increases, user engagement increases, and churn rates decline.
Understanding Local Data Storage in Flutter
1. Shared Preferences
What it is: A lightweight key-value storage for very small amounts of data.
Best For: User preferences, app settings, and basic data types, such as strings, integers, and booleans.
Use Case: For example, user login status, theme settings, or recently viewed pages can be saved.
2. SQLite Database
What it is: This is a structured relational database that facilitates efficient data storage, querying, and management. Why is this?
Suitable for: Devices with complex data structures or extensive datasets that necessitate persistent storage.
Use: Maintaining user information, online product listings, or keeping track of local tasks.
3. File System Storage
What it is: Great for Flutter applications that read and write files straight in local storage on devices.
Suitable for: Saving large files such as images, videos, and audio.
Use Case: Media offline storage for streaming applications or downloaded content.
4. NoSQL Databases
What's It: Schema-less and fast read/write storage solutions such as Hive and Drift (Moor).
Suitable For: Applications that need scale very much, have high performance, and fast access to data.
Use Case: Store User Profile, App Cache, Logs.
Choosing the Right Local Storage Solution
However, if there are general methods of storage you will want to keep in mind:
Shared Preferences: For lightweight and relatively simple data, such as user settings.
SQLite: For relational and structured data that is expected to be queried in complex ways.
File System Storage: Good for large files such as images and videos or those you want to download for offline access.
NoSQL Databases: When focusing on flexibility, performance, and scalability.
Step-by-Step Guide to Implementing Offline Functionality in Flutter
1. Adding SQLite for Data Persistence
Step 1: Add dependencies to pubspec.yaml.
dependencies:
sqflite: ^2.0.0
path_provider: ^2.0.0
Step 2: Create a Database Helper Class.
import 'package:sqflite/sqflite.dart';
import 'package:path_provider/path_provider.dart';
import 'dart: io';
class DatabaseHelper {
static Database? _database;
Future<Database> get database async {
if (_database != null) return _database!;
_database = await _initDB();
return _database!;
}
_initDB() async {
Directory documentsDirectory = await getApplicationDocumentsDirectory();
String path = '${documentsDirectory.path}/my_database.db';
return await openDatabase(path, version: 1, onCreate: _onCreate);
}
void _onCreate(Database db, int version) async {
await db.execute('CREATE TABLE Users (id INTEGER PRIMARY KEY, name TEXT)');
}
}
2. Implementing Shared Preferences for Key-Value Storage
Step 1: Add dependencies
dependencies:
shared_preferences: ^2.0.0
Step 2: Store and retrieve data
import 'package:shared_preferences/shared_preferences.dart';
Future<void> savePreference(String key, String value) async {
final prefs = await SharedPreferences.getInstance();
await prefs.setString(key, value);
}
Future<String?> getPreference(String key) async {
final prefs = await SharedPreferences.getInstance();
return prefs.getString(key);
}
Caching Network Data for Offline Use
To cache network responses for offline access, use libraries like dio with dio_http_cache or write responses to the local database.
Example with Dio
dependencies:
dio: ^4.0.0
dio_http_c
import 'package:dio/dio.dart';
import 'package:dio_http_cache/dio_http_cache.dart';
final dio = Dio();
Dio.interceptors.add(DioCacheManager(CacheConfig()).interceptor);
Future fetch data() async {
final response = await dio.get(
'https://example.com/api',
options: buildCacheOptions(Duration(days: 7)),
);
}
Best Practices for Offline App Development in Flutter
Design Offline-First: Create the application while assuming no access to the internet at all.
Provide Feedback: Let there be visual indications for the state of connection and the offline capability.
Data Validation: All validation and cleansing operations would take place at the local end before syncing them.
Sync Conflict Resolution: Implement a strategy for solving conflicts.
Optimize Performance: Limit storage footprints and make sure they optimize caching.
Future Trends in Offline Data Management
PWA: Offline use and services are made possible through service workers.
Edge Computing: Faster offline processes in comparison to servers.
P2P Sharing: Allows data to be shared without contacts between devices.
Intelligent Caching: State-of-the-art techniques for prefilling and caching of data.